Tom Freehill
Game Developer
Engine of Evil (written in C++)
-
Engine: built a 2D isometric game engine from the ground up using SDL2 for hardware.
-
Resource Management: separated design-time elements to dynamically loaded external files.
-
Renderer: created a custom GPU-bound render pipeline optimized for 60-1000+ FPS when drawing large, complex, moving scenes.
-
Collision: designed a collision system that detects and seamlessly resolves 2D collisions.
-
AI: developed a custom non-A* navigation system that can operate on many entities at once.
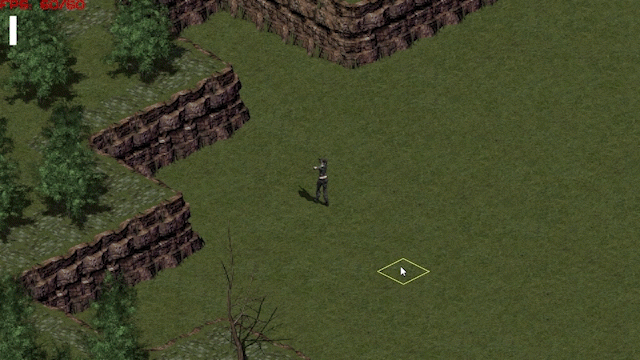
The input system is flexible enough to support multiple player control impementations. These are three examples.
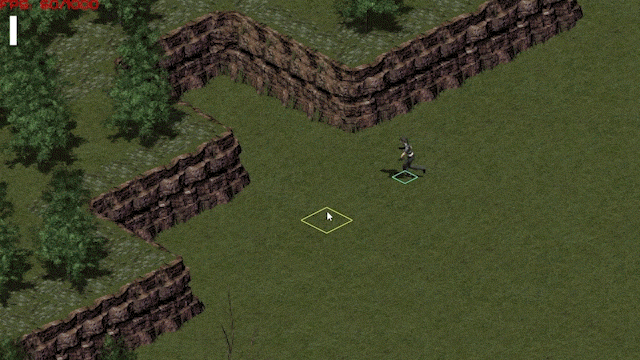
Static-Dynamic and Dynamic-Dynamic collisions are resolved by shortest-path de-penetration and redirecting the remaining velocity vector. A color-coded debug view of colliders simplifies correcting any runtime issues.
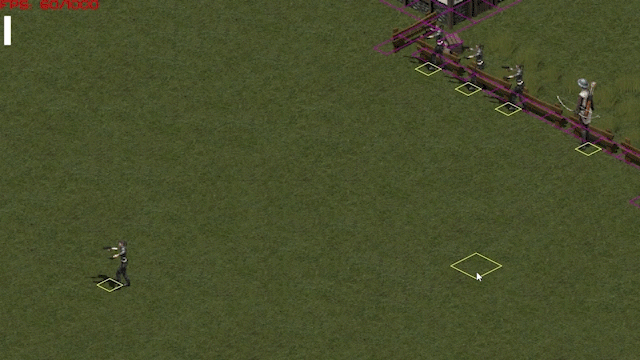
An AI can efficiently navigate its dynamic collider around other dynamic colliders en-route to its next waypoint. It leverags the spatially partitioned collision world, broad-phase tests, raycasts, boxcasts, and narrow-phase collision tests.
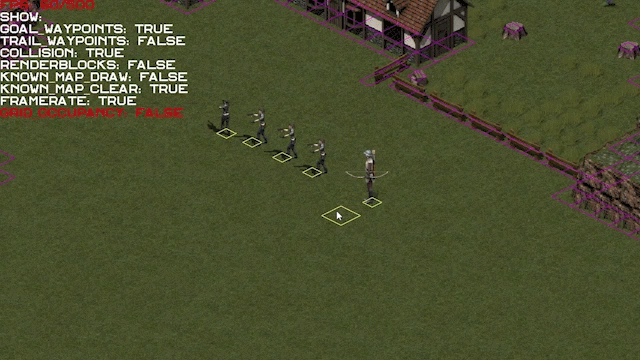
The renderer supports frustum culling, pan, zoom, and alpha blending of all textures. The collision system supports 2D AABB and OBB colliders, the details and placement of which are loaded from text files populated at design-time.
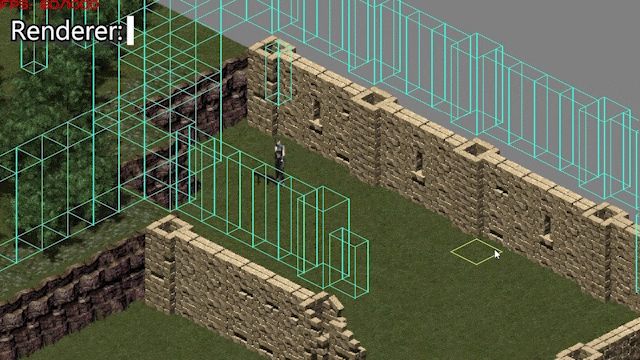
Each tile and sprite is given a true-3D bounding box, which occupies cells of a spatially partitioned render-world. Perspective-sorting these 3D bounds solves common issues with rendering 2D isometric tilemaps. For example, this engine can draw sprites taller and/or wider than one tile, and correctly sorts stacks of tiles across multiple layers.
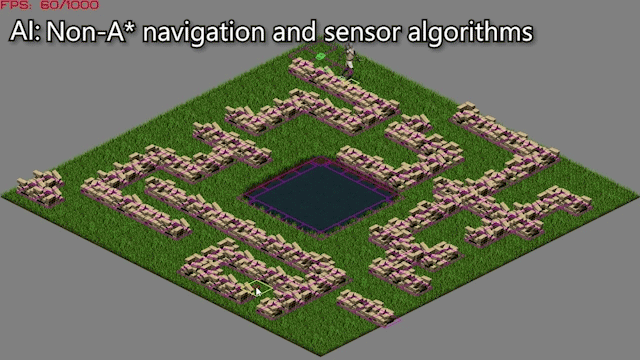
The navigation system uses collision sensors to detect walls and corners, raycast and boxcasts to avoid collision, a memory of visited cells to avoid useless vectors, breadcrumbs for backtracking, and several heuristics all in service of reaching the next waypoint.
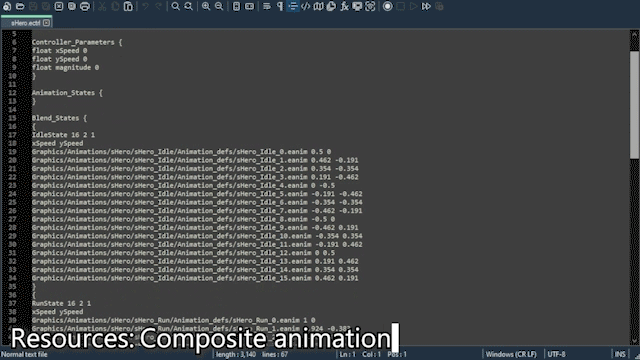
Animation Controller files can be built at design-time and loaded dynamically. They use texture and animation files to define a state machine which selects and plays sprite frames based on atomic control parameters, and state transitions. Animation Controllers support both simple animation states, and composite animation blend states.

Entities that appear in a game world are loaded from unique prefab files. They can have all, some, or none of the listed components. For example, an entity can have a static (non-animated) sprite and collision, but no AI, or player input. Or, an entity can have all of the above.
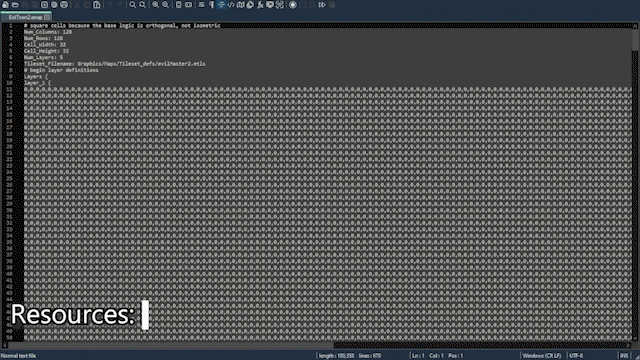
An Engine of Evil map file brings together all design-time elements and files into a single file. It defines how the tileset will be laid out in the game world, where the entities will be placed, and how the collision and render worlds will be partitioned.
Quake 2: Soul Collector Mod (written in C)
-
AI: integrated a framework that allows the player to control any in-game monster.
-
Camera: programmed a third-person view that responds to player control and collision.
-
HUD/UI: incorporated new inventory and control systems that the player can view at runtime.
-
Engine: improved the sound management to account for an unlimited number of sounds.
-
Audio/Art: added new user-friendly sounds and art to indicate added game functions.
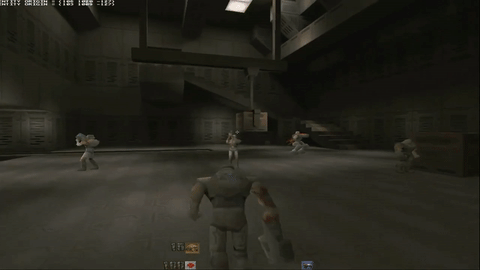
The player controls its host to move, attack, and give commands to other enemies.
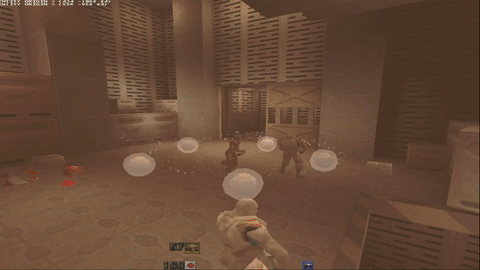
Soul Collector can be played in single player and multiplayer cooperative with all the same abilities. Player one has left his body (right) and is watching player two (left) run around with a damage-absorbing shield of souls.
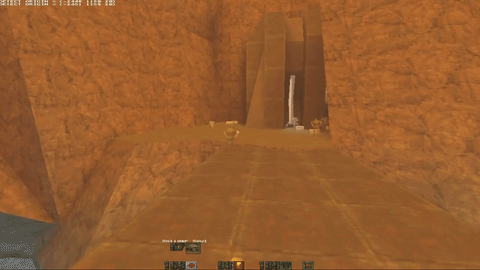
When enemies die they leave behind ghostly wailing souls only visible while the player is a ghost. The player is using a magnetic pull effect here to collect several souls.
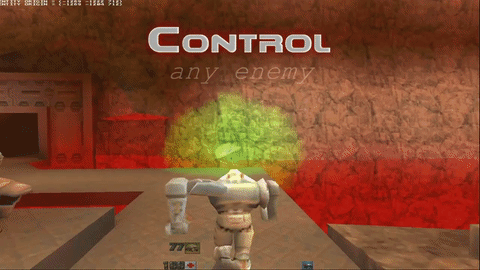
All enemy types can be controlled in this mod, including those that swim or fly. This shows one running, and another swimming, towards user-defined goals indicated by the green item.


As the player collects souls, new abilities are earned at fixed thresholds. For example, the player first earns the ability to possess and control enemies at level 2 after collecting 5 souls.

The control scheme matching the player's current status can be displayed by pressing F7. For example, a player controlling an enemy precisely will see the "Uber Host" controls, and upon returning to their own body will see the "Corporeal" controls.

A new sound management protocol removes all cached sounds that no longer appear in a level, and prevents fragmentation of the sounds array when caching new sounds. This allows for a near-unlimited number of sounds per level.
Halt! Catch Fire! (written in C#)
-
AI: designed and coded optimized high-volume A* pathfinding and finite state machines.
-
HUD/UI: programmed level intermissions and endgame, all HUD elements, and highscore save system.
-
Gameplay: took ownership of the design and implementation of all mechanics.
-
Management: coordinated and led a team of seven (7) during a local month-long game jam.
-
Documentation: performed a daily scrum to track progress and maintain a focused scope.
-
Playtesting: presented the game at local events, and improved the game through playtesters’ feedback.
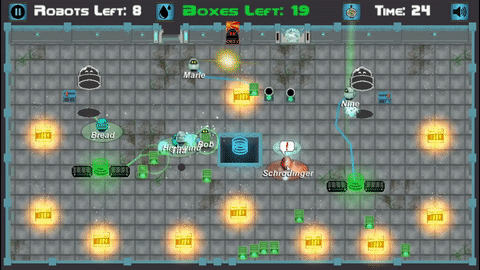
Hectic action characterizes Halt! Catch Fire! While robots collect all-important boxes, they must survive electrical explosions, gravitational implosions, fires, pits, crushers, and each other with the player's help.

After each level the player is given a performance review and the next part of the story.

The player is able to globally mute, adjust individual sound channels, start a new game, or quit at all times (especially useful to mobile users).

After beating the game or getting a game over with a qualifying top-5 score, players can enter a custom high score.
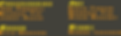
Credit where credit is due.

Every robot has this finite state machine. The "Chase State" tells the robot how to pursue and grab its current target. The "Search State" tells the robot how to find a target. Having a target dictates transitions between these overall states.

The finite state machine of each robot can be interrupted and resolved at any point if any of the shown conditions are met. Blue and red indicate a suicidal and homicidal robot's unique extra interrupts, respectively.
Doom: Gated Community (Final Doom PWAD)
-
AI: utilized AI behavior to procedurally generate unique combat encounters and map traversal.
-
Level Design: built a fast-paced map focused on combat tactics and immersive story.
-
Gameplay: maximized replay value with balanced combat generation and detailed content exploration.
-
Testing: playtested hundreds of map iterations using the Doom Legacy source port

The level is littered with artifacts and structures that let players discover its story.

The player has limited access to teleports, however the entire level is designed for quick movement and flow to any room.
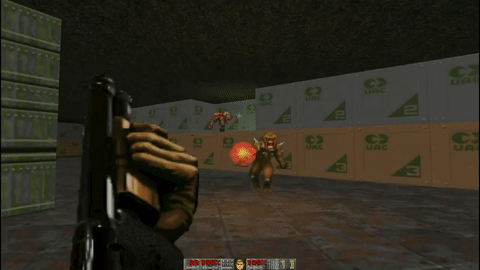
Using the network, monsters can teleport defensively and offensively.

A single yellow key that lets the player beat the level appears in a random location each playthrough.

A pool of monsters (top) floods into the level by a randomizing network of teleports (right, and throughout). A player's movement through the map triggers capture-and-release mechanisms; these feed monsters back through the distribution system, while focusing its output on the player's location and adjacent areas.

Quake 4: Black Thunder Stealth Mod (written in C++)
-
Gameplay: designed six (6) new stealth mechanics in-line with the fast-paced shooter action of Quake 4.
-
HUD/UI: integrated player illumination tracking, spell mana management, and third-person crosshairs.
-
Physics: coded projectiles that bind to physics joints and world geometry, and projectiles that regulate ragdoll physics activation/deactivation.
-
AI: extended AI behavior to include player-triggered entity spawning, and impaired player-tracking based on visible light sources.
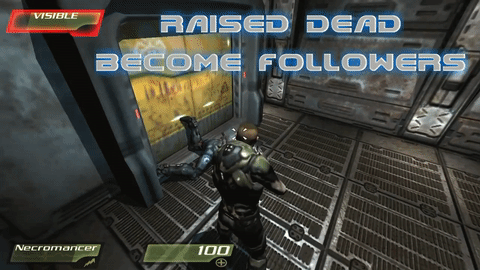
The lightning gun can toggle from doing damage to giving life. An unlimited number of raised dead follow and defend the player.

Raising the dead costs mana over time. When all mana for a spell is spent, it begins to regnerate, seen here in the highlighted box. During a spell's mana regeneration the player is unable to use that spell.
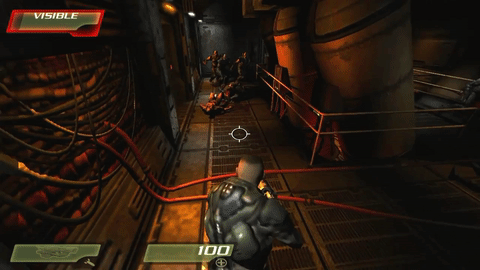
Paralysis bombs emit smoke that ragdolls enemies within a fixed radius. After the gas clears and a few seconds pass enemies resume fighting. Paralysis does not affect teammates or followers.

Sticky bombs attach to any surface they contact, including actors' body parts, and world geometry. The bombs here are the small cylinders with two red stripes on them.

The player chooses when to detonate all sticky bombs simultaneously by pressing 'b'.
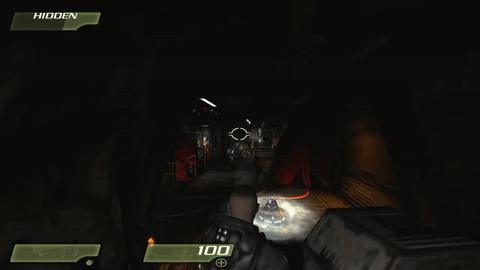
The portal gun teleports any actor that touches one portal (glowing white) to the other portal. In this example, a strogg has a sticky bomb attached to it when it teleports, then the player remote-detonates it down the hall.
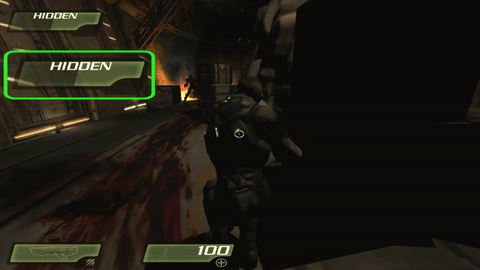
Player illumination is calculated from the number, type, and intensity of lights that have line-of-sight to the player. Below 50% illumination disallows enemies from tracking beyond the player's last known location.
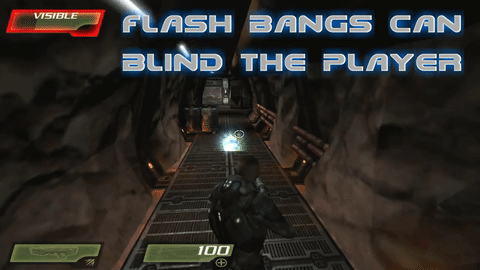
Flash bangs that detonate in an AI's field of view (fov) remove all its enemies and prevent it from tracking the player for 20 seconds. Detonations in the player's fov also temporarily blind the player, as seen here.